Hello, In this post we will learn about mysqli query to insert data into database using HTML form. For this process we have to required 2 files, one is HTML file and another is php file. HTML file is used to create a HTML form and php file is used to define database connection or insert query.
Here i created a simple HTML form that have <input> text field and submit field to trigger insert query.
index.html
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Add User Record Form</title> </head> <body> <form action="insert.php" method="post"> <p> <label for="firstName">First Name:</label> <input type="text" name="first_name" id="firstName"> </p> <p> <label for="lastName">Last Name:</label> <input type="text" name="last_name" id="lastName"> </p> <p> <label for="email">Email Address:</label> <input type="text" name="email" id="email"> </p> <p> <label for="phone">Phone:</label> <input type="text" name="phone" id="phone"> </p> <input type="submit" name="submit" value="Submit"> </form> </body> </html> |
From above HTML form, when user fill the fields detail and click on submit button, the form data will passed to “insert.php” file. The ‘insert.php‘ file create the connection to MYSQL Database and retrieves form fields data using $_POST variable, after this trigger the insert query to add record. Below is the complete code of ‘insert.php‘ file.
insert.php
MYSQLi Procedural Interface
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
<?php // create database connection $host="localhost"; $username="root"; $password=""; $database="test"; $con=mysqli_connect($host,$username,$password,$database); //test connection if($con!=true){ die("Database connection error:".mysqli_connect_error()); } // Retrieves the form data usig $_POST method if($_REQUEST['submit']){ $first_name=$_POST['first_name']; $last_name=$_POST['last_name']; $email=$_POST['email']; $phone=$_POST['phone']; $sql="INSERT INTO user (first_name,last_name,email,phone) VALUES ('$first_name','$last_name','email','$phone')"; $result=mysqli_query($con,$sql); if($result){ echo "New Record Inserted Successfully"; }else{ echo "Error, Record not inserted."; } } mysqli_close($con); ?> |
MYSQLi Object-oriented Interface
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
<?php // create database connection $host="localhost"; $username="root"; $password=""; $database="test"; $con=new mysqli($host,$username,$password,$database); //test connection if($con->connect_error){ die("Database connection error:".$con->connect_error); } // Retrieves the form data usig $_POST method if($_REQUEST['submit']){ $first_name=$_POST['first_name']; $last_name=$_POST['last_name']; $email=$_POST['email']; $phone=$_POST['phone']; $sql="INSERT INTO user (first_name,last_name,email,phone) VALUES ('$first_name','$last_name','email','$phone')"; $result=$con->query($sql); if($result){ echo "New Record Inserted Successfully"; }else{ echo "Error, Record not inserted."; } } $con->close(); ?> |
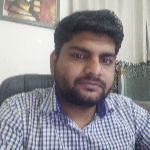
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website”. He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone, e27.co