When displaying dates and times on websites, it’s often more user-friendly to show a relative time, such as “5 minutes ago” or “2 days ago,” rather than a specific timestamp like “2024-08-01 14:00:00”. This format gives users a better sense of how recent an event was. PHP makes it easy to Convert a Timestamp to a “Time Ago” format with a custom function.
Why Convert a Timestamp to a “Time Ago” Format?
- User Experience: It’s easier for users to understand “2 hours ago” compared to “2024-08-01 14:00:00”.
- Relevance: “Time ago” format keeps content looking fresh and current.
- Engagement: Users are more likely to engage with content that appears recent.
Suggested Read: How to generate dynamic QR code using PHP
Converting a Timestamp to a “Time Ago” Function in PHP
Let’s start by creating a PHP function that takes a timestamp as an input and returns a string representing the “time ago” format.
Step 1: Calculate the Time Difference
First, we need to find the difference between the current time and the timestamp provided.
2 3 4 5 6 7 8 9 10 |
function timeAgo($timestamp) { $timeAgo = time() - $timestamp; if ($timeAgo < 1) { return 'just now'; } } |
'time()
returns the current Unix timestamp.
- Subtracting the provided timestamp from the current time gives the difference in seconds.
Step 2: Define Time Units
Next, we define the various time units (seconds, minutes, hours, days, etc.) and how many seconds are in each unit.
2 3 4 5 6 7 8 9 10 11 12 |
$timeUnits = [ 31536000 => 'year', 2592000 => 'month', 604800 => 'week', 86400 => 'day', 3600 => 'hour', 60 => 'minute', 1 => 'second' ]; |
- Each key represents the number of seconds in a given unit.
- The corresponding value is the name of the time unit.
Step 3: Calculate the Largest Unit
We loop through each time unit to find the largest one that fits within the time difference.
2 3 4 5 6 7 8 9 |
foreach ($timeUnits as $unitSeconds => $unitName) { if ($timeAgo >= $unitSeconds) { $unitValue = floor($timeAgo / $unitSeconds); return $unitValue . ' ' . $unitName . ($unitValue > 1 ? 's' : '') . ' ago'; } } |
floor($timeAgo / $unitSeconds)
` calculates how many full units fit into the time difference.- If the result is greater than or equal to 1, we return that value along with the appropriate time unit name.
- If the unit value is greater than 1, we pluralize the unit name (e.g., “2 days ago”).
Are you want to get implementation help, or modify or extend the functionality?
A Tutorialswebsite Expert can do it for you.
Complete Function Code
Here’s the complete function that you can use in your PHP projects:
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
function timeAgo($timestamp) { $timeAgo = time() - $timestamp; if ($timeAgo < 1) { return 'just now'; } $timeUnits = [ 31536000 => 'year', 2592000 => 'month', 604800 => 'week', 86400 => 'day', 3600 => 'hour', 60 => 'minute', 1 => 'second' ]; foreach ($timeUnits as $unitSeconds => $unitName) { if ($timeAgo >= $unitSeconds) { $unitValue = floor($timeAgo / $unitSeconds); return $unitValue . ' ' . $unitName . ($unitValue > 1 ? 's' : '') . ' ago'; } } } |
How to Use the Function
Using the timeAgo
function is simple. Just pass in a Unix timestamp, and it will return the “time ago” string.
Example Usage
2 3 4 5 |
$timestamp = strtotime('2024-09-03 20:36:00'); // Example timestamp echo timeAgo($timestamp); |
Output Examples:
- If the timestamp was 5 minutes ago, it would return
"5 minutes ago"
. - If it was 2 days ago, it would return
"2 days ago"
. - If it was just seconds ago, it would return
"just now"
.
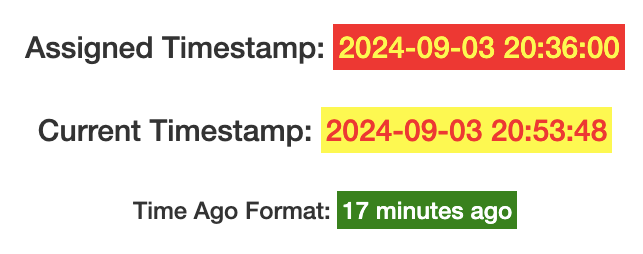
A Few Things to Keep in Mind
While this function covers most cases, here are a couple of things to think about:
- Future Timestamps: Right now, if you pass a future timestamp, it returns “just now.” You might want to add a check to handle future dates differently.
- Localization: If your site supports multiple languages, you might want to translate the time units.
Do you want to get implementation help, or modify or extend the functionality of this script? Submit a paid service request
Conclusion
Converting a timestamp to a “time ago” format in PHP is a simple yet powerful way to improve the user experience on your website. With just a bit of code, you can make your content more relatable and engaging for your users.
Feel free to use and modify this function to suit your needs. It’s a handy tool that can make your site feel a lot more dynamic!
Also Read: Convert a Timestamp to a “Time Ago” Format
FAQs
The “time ago” format displays time in a relative way, such as “5 minutes ago” or “2 days ago,” instead of an exact timestamp. It helps users quickly understand how recent an event is.
To convert a timestamp, you can use a PHP function that calculates the difference between the current time and the timestamp, then formats it into a human-readable string like “3 hours ago.”
The provided function currently returns “just now” for future timestamps. You can modify the function to handle future dates as needed, such as displaying “in the future.”
Yes, the timeAgo
function is compatible with PHP 5.3 and later. Ensure your PHP version supports the functions used in the code.
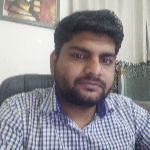
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website”. He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone, e27.co