Write a function to find the second largest element in an array. The function takes an array of integers given a string as an argument and returns the second max value from the input array. If there is no second max return -1.
Also Read: PHP Array Function
Let’s take some examples:
2 3 4 5 6 7 8 9 10 11 |
1. For array ["3", "-2"] should return "-2" 2. For array ["5", "5", "4", "2"] should return "4" 3. For array ["4", "4", "4"] should return "-1" (duplicates are not considered as the second max) 4. For [] (empty array) should return "-1". |
Find the second largest element in an array
Simple Solution
The idea is to sort the array in descending order and then return the second element which is not equal to the largest element from the sorted array. Array value will be entered from the user using the input text.
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 |
import React, {useState} from "react"; //import logo from './logo.svg'; import './App.css'; function App() { let [result, setResult] = useState(''); let [arr, setArr] = useState([]); const title="Find the second largest element"; function saveInput(input) { let newArr=[...arr]; let length=newArr.length; console.log(length+' : '+newArr); newArr[length]=input; setArr(newArr); console.log(length+' : '+newArr); }; function findSecondLagestNumber(arr=[]){ arr = arr.sort((a, b) => (parseInt(b) - parseInt(a))); if(arr.length){ let arr_size= arr.length / arr[0].length; setResult(print2largest(arr, arr_size)); }else{ setResult("-1"); } function print2largest(arr, arr_size){ let i=''; let array_size=arr_size; /* There should be atleast two elements */ if (array_size <= 2) { array_size = array_size + 2; } console.log(array_size); for (i = array_size-2 ; i >= 0; i--) { // if the element is not // equal to largest element if (arr[i] !== arr[array_size - 1]) { console.log(arr[i]) return arr[i]; } } return "-1"; } } function refreshPage() { window.location.reload(false); } return ( <div className="App"> <div>{title} </div> <h4>Total Input Value :{arr}</h4> <h4>{result}</h4> <input type="text" onBlur={(e)=>saveInput(e.target.value)} /><br></br> Click outside the input box to store input value in array <br></br> <br></br> <button onClick={()=>findSecondLagestNumber(arr)}> Click second largest element</button> <br></br> <br></br> <button onClick={()=>refreshPage()}> Reset</button> </div> ); } export default App; |
Input array value: array [“5”, “5”, “4”, “2”]
Output:
2 3 4 |
The second largest element is 4. |
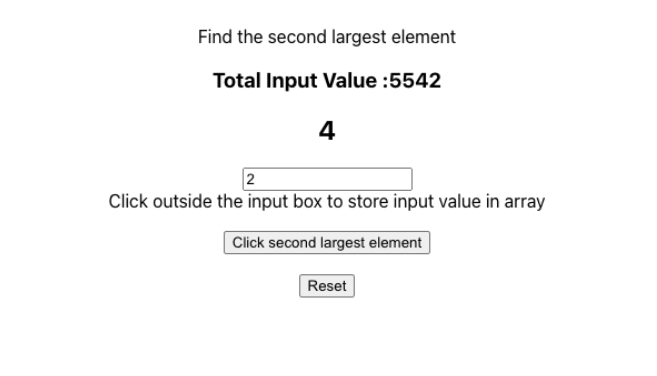
Complexity Analysis:
- Time Complexity: O(n log n).
Time required to sort the array is O(n log n). - Auxiliary space: O(1).
As no extra space is required.
Also Read: How to convert array data into Simple XML file using PHP
Conclusion
Thank you for reading Find the second largest element in an array using React article. Hope I was able to help someone out. If you have any questions feel free to ask anything using the contact form. Cheers!!.
Are you want to get implementation help, or modify or extend the functionality of this script? Submit paid service request
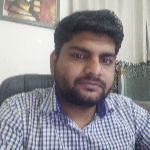
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website”. He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone, e27.co