PHP Text Captcha is a image of text which is generated by free captcha script. It is used to protect your login form, register form or any forms from spam ,abuse and hackers.
In this article, we will learn How to create PHP Text Captacha without google captcha API. PHP Text Captcha is open source script which is used to create complex images.
Features of PHP Text Captcha:
- Code Structure is small.
- phpTextClass is used to create captcha image
- Code is fully customizable
- Security features with random lines and noise
- Protection from spammer and abuse script.
- Remove possibility to hack information from random hackers script.
phpcaptchaClass.php
In this file, we create a phpcaptcha class , hexTo RGB and ImageTTFCenter function to create imgae of text captcha.
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 |
<?php /*phpcaptcha class, version 1.0 created by www.tutorialswebsite.com (Pradeep Maurya) october 5, 2017 */ class phpcaptchaClass { public function phptext($text,$textColor,$backgroundColor='',$fontSize,$imgWidth,$imgHeight,$dir,$fileName) { /* settings */ $font = './calibri.ttf';/*define font*/ $textColor=$this->hexToRGB($textColor); $im = imagecreatetruecolor($imgWidth, $imgHeight); $textColor = imagecolorallocate($im, $textColor['r'],$textColor['g'],$textColor['b']); if($backgroundColor==''){/*select random color*/ $colorCode=array('#56aad8', '#61c4a8', '#d3ab92'); $backgroundColor = $this->hexToRGB($colorCode[rand(0, count($colorCode)-1)]); $backgroundColor = imagecolorallocate($im, $backgroundColor['r'],$backgroundColor['g'],$backgroundColor['b']); }else{/*select background color as provided*/ $backgroundColor = $this->hexToRGB($backgroundColor); $backgroundColor = imagecolorallocate($im, $backgroundColor['r'],$backgroundColor['g'],$backgroundColor['b']); } imagefill($im,0,0,$backgroundColor); list($x, $y) = $this->ImageTTFCenter($im, $text, $font, $fontSize); imagettftext($im, $fontSize, 0, $x, $y, $textColor, $font, $text); if(imagejpeg($im,$dir.$fileName,90)){/*save image as JPG*/ return json_encode(array('status'=>TRUE,'image'=>$dir.$fileName)); imagedestroy($im); } } public function phpcaptcha($textColor,$backgroundColor,$imgWidth,$imgHeight,$noiceLines=0,$noiceDots=0,$noiceColor='#162453') { /* Settings */ $text=$this->random(); $font = './font/monofont.ttf';/* font */ $textColor=$this->hexToRGB($textColor); $fontSize = $imgHeight * 0.75; $im = imagecreatetruecolor($imgWidth, $imgHeight); $textColor = imagecolorallocate($im, $textColor['r'],$textColor['g'],$textColor['b']); $backgroundColor = $this->hexToRGB($backgroundColor); $backgroundColor = imagecolorallocate($im, $backgroundColor['r'],$backgroundColor['g'],$backgroundColor['b']); /* generating lines randomly in background of image */ if($noiceLines>0){ $noiceColor=$this->hexToRGB($noiceColor); $noiceColor = imagecolorallocate($im, $noiceColor['r'],$noiceColor['g'],$noiceColor['b']); for( $i=0; $i<$noiceLines; $i++ ) { imageline($im, mt_rand(0,$imgWidth), mt_rand(0,$imgHeight), mt_rand(0,$imgWidth), mt_rand(0,$imgHeight), $noiceColor); }} if($noiceDots>0){/* generating the dots randomly in background */ for( $i=0; $i<$noiceDots; $i++ ) { imagefilledellipse($im, mt_rand(0,$imgWidth), mt_rand(0,$imgHeight), 3, 3, $textColor); }} imagefill($im,0,0,$backgroundColor); list($x, $y) = $this->ImageTTFCenter($im, $text, $font, $fontSize); imagettftext($im, $fontSize, 0, $x, $y, $textColor, $font, $text); imagejpeg($im,NULL,90);/* Showing image */ header('Content-Type: image/jpeg');/* defining the image type to be shown in browser widow */ imagedestroy($im);/* Destroying image instance */ if(isset($_SESSION)){ $_SESSION['captcha_code'] = $text;/* set random text in session for captcha validation*/ } } /*for random string*/ protected function random($characters=6,$letters = '23456789bcdfghjkmnpqrstvwxyz'){ $str=''; for ($i=0; $i<$characters; $i++) { $str .= substr($letters, mt_rand(0, strlen($letters)-1), 1); } return $str; } /*function to convert hex value to rgb array*/ protected function hexToRGB($colour) { if ( $colour[0] == '#' ) { $colour = substr( $colour, 1 ); } if ( strlen( $colour ) == 6 ) { list( $r, $g, $b ) = array( $colour[0] . $colour[1], $colour[2] . $colour[3], $colour[4] . $colour[5] ); } elseif ( strlen( $colour ) == 3 ) { list( $r, $g, $b ) = array( $colour[0] . $colour[0], $colour[1] . $colour[1], $colour[2] . $colour[2] ); } else { return false; } $r = hexdec( $r ); $g = hexdec( $g ); $b = hexdec( $b ); return array( 'r' => $r, 'g' => $g, 'b' => $b ); } /*function to get center position on image*/ protected function ImageTTFCenter($image, $text, $font, $size, $angle = 8) { $xi = imagesx($image); $yi = imagesy($image); $box = imagettfbbox($size, $angle, $font, $text); $xr = abs(max($box[2], $box[4])); $yr = abs(max($box[5], $box[7])); $x = intval(($xi - $xr) / 2); $y = intval(($yi + $yr) / 2); return array($x, $y); } } ?> |
index.php
This file is used to create a form data and used to validate captcha image after submitting the form.
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
<?php session_start(); if(isset($_POST['Submit'])){ // code for check server side validation if(empty($_SESSION['captcha_code'] ) || strcasecmp($_SESSION['captcha_code'], $_POST['captcha_code']) != 0){ $msg="<span style='color:red'>The Validation code does not match!</span>";// Captcha verification is incorrect. }else{// Captcha verification is Correct. Final Code Execute here! $msg="<span style='color:green'>The Validation code has been matched.</span>"; } } ?> <!doctype html> <html> <head> <meta charset="utf-8"> <title>PHP Secure text Captcha.</title> <link href="./css/style.css" rel="stylesheet"> <script type='text/javascript'> function refreshCaptcha(){ var img = document.images['captchaimg']; img.src = img.src.substring(0,img.src.lastIndexOf("?"))+"?rand="+Math.random()*1000; } </script> </head> <body> <div id="frame0"> <center> <h1>PHP Secure Text Captcha Demo.</h1> <p>More tutorials <a href="https://www.tutorialswebsite.com">www.tutorialswebsite.com</a></p></center> </div> <br> <form action="" method="post" name="form1" id="form1" > <table width="400" border="0" align="center" cellpadding="5" cellspacing="1" class="table"> <?php if(isset($msg)){?> <tr> <td colspan="2" align="center" valign="top"><?php echo $msg;?></td> </tr> <?php } ?> <tr> <td align="right" valign="top"> Validation code:</td> <td><img src="captcha.php?rand=<?php echo rand();?>" id='captchaimg'><br> <label for='message'>Enter the code above here :</label> <br> <input id="captcha_code" name="captcha_code" type="text"> <br> Can't read the image? click <a href='javascript: refreshCaptcha();'>here</a> to refresh.</td> </tr> <tr> <td> </td> <td><input name="Submit" type="submit" onclick="return validate();" value="Submit" class="button1"></td> </tr> </table> </form> </body> </html> |
captcha.php
This file is used to create captcha image. In this file, we include the “phpcaptchaClass.php” file and create an object of phpcaptchaClass.
phpcaptcha():- this function create image with text and lines or noise based on parameter.
2 3 4 5 6 7 8 9 10 11 12 |
<?php session_start(); include("./phpcaptchaClass.php"); /*create class object*/ $phptextObj = new phpcaptchaClass(); /*phptext function to genrate image with text*/ $phptextObj->phpcaptcha('#000','#fff',120,40,10,25); ?> |
Conclusion
In the example script, the static images are showing on the 3D images gallery.
Are you want to get implementation help, or modify or extend the functionality of this script? Submit paid service request
[sociallocker]
[/sociallocker]
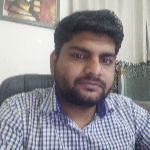
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website”. He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone, e27.co