It might be quite useful to get geolocation data based on IP addresses if you’re developing a PHP application and want to display location-based content, track users’ regions, or analyse geographic data. This post will show several ways to retrieve geolocation from an IP address in PHP, including the use of downloadable databases and APIs.
Suggested Read: How to Get Current Visitor Location using HTML5 Geolocation API
Why Use IP-Based Geolocation?
Geolocation based on IP allows you to:
- Use a user’s location to personalise content.
- Make relevant location suggestions to enhance the user experience (e.g., by choosing a default currency or country).
- For analytics or marketing purposes, analyse traffic trends.
Get the IP Address of the Current User
To get the current user’s IP address in PHP, access it using $_SERVER['REMOTE_ADDR']
.
2 3 4 |
$current_ip = $_SERVER["REMOTE_ADDR"]; |
To get the location of a specific IP address, assign it to the $current_ip
variable.
2 3 4 |
$current_ip = "152.59.172.230"; |
Methods to Get Geolocation in PHP
1. Using an IPinfo Geolocation API
APIs are a straightforward solution if you don’t need to store the geolocation data locally. They provide the data on-demand and are often easy to implement.
IPinfo is a popular API for retrieving geolocation data from an IP address. Here’s how to set it up in PHP:
- Sign Up for an API Key: Register on IPinfo’s website to get an API token.
- Create a PHP Function: Use cURL or file_get_contents to make a request to IPinfo’s API.
Here’s a sample PHP function to retrieve location data:
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
<?php function getGeolocation($ip) { $apiKey = 'YOUR_IPINFO_API_KEY'; $url = "http://ipinfo.io/{$ip}?token={$apiKey}"; $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1); $response = curl_exec($curl); curl_close($curl); $data = json_decode($response, true); return $data; } // Example Usage $current_ip = $_SERVER["REMOTE_ADDR"]; //'152.59.172.230' Replace with the user's IP $locationData = getGeolocation($current_ip); print_r($locationData); |
This will give you data like the city, region, country, and coordinates.
Output:
2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
Array ( [ip] => 152.59.172.230 [city] => Varanasi [region] => Uttar Pradesh [country] => IN [loc] => 25.3167,83.0104 [org] => AS55836 Reliance Jio Infocomm Limited [postal] => 221001 [timezone] => Asia/Kolkata ) |
Are you want to get implementation help, or modify or extend the functionality of this script?
A Tutorialswebsite Expert can do it for you.
2. Using the IPstack API
The IPstack API is another popular service with a large range of data, including ISP, location, and currency information.
- Sign Up for IPstack and get an access key.
- Send an API Request in PHP
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
<?php function getGeolocationIpstack($ip) { $accessKey = 'YOUR_IPSTACK_API_KEY'; $url = "http://api.ipstack.com/{$ip}?access_key={$accessKey}"; $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1); $response = curl_exec($curl); curl_close($curl); $data = json_decode($response, true); return $data; } // Example Usage $current_ip = $_SERVER["REMOTE_ADDR"]; //'152.59.172.230' Replace with the user's IP $locationData = getGeolocationIpstack($current_ip); print_r($locationData); |
This will yield similar information to IPinfo, with additional data points based on your subscription plan.
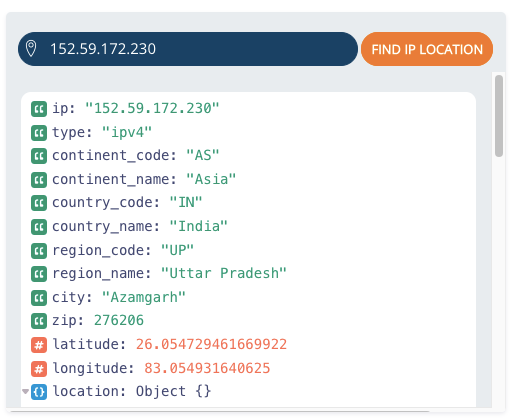
3. Using MaxMind’s GeoLite2 Database
If you want to avoid API calls, you can download the MaxMind GeoLite2 database, a free database that you can host locally. This method is ideal for applications with high traffic or strict privacy requirements since you store the data yourself.
- Download GeoLite2-City database: Get it from MaxMind’s website.
- Install the GeoIP2 PHP Library:
Run this command to install the required library via Composer:
2 3 4 |
composer require geoip2/geoip2 |
- Use the Database in PHP
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
<?php require 'vendor/autoload.php'; use GeoIp2\Database\Reader; function getGeolocationMaxMind($ip) { $reader = new Reader('/path/to/GeoLite2-City.mmdb'); try { $record = $reader->city($ip); return [ 'network' => $record->traits->network, 'country_code' => $record->country->isoCode, 'country_name' => $record->country->name, 'city' => $record->city->name, 'state' => $record->mostSpecificSubdivision->name, 'postal_code' => $record->postal->code, 'country' => $record->country->name, 'latitude' => $record->location->latitude, 'longitude' => $record->location->longitude ]; } catch (Exception $e) { return ['error' => 'Location not found']; } } // Example Usage $current_ip = $_SERVER["REMOTE_ADDR"]; //'152.59.172.230' Replace with the user's IP $locationData = getGeolocationMaxMind($current_ip); print_r($locationData); |
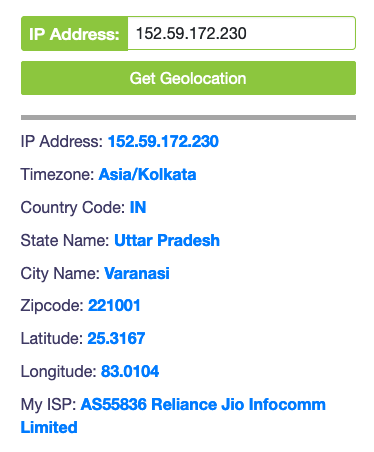
Are you want to get implementation help, or modify or extend the functionality of this script?
A Tutorialswebsite Expert can do it for you.
Choosing the Right Solution
- APIs like IPinfo and IPstack are best for lightweight and quick implementations, ideal for lower traffic websites and applications.
- Self-hosted databases like MaxMind’s GeoLite2 are suitable for high-traffic sites or those requiring offline data storage without dependency on an external API.
Each option has unique benefits and constraints, so consider factors like traffic volume, budget, and data accuracy before deciding.
Are you want to get implementation help, or modify or extend the functionality of this script? Submit a paid service request
Conclusion
Geolocation data from IP addresses can be rapidly retrieved in PHP using APIs or databases hosted by MaxMind, such as GeoLite2. APIs are simple to set up, however they may include request restrictions or fees for heavy usage. A local database solution allows for greater flexibility and privacy control, but it requires regular upgrades. Hopefully, this post helped you identify the best way for get IP-based geolocation in PHP!
Also Read: How to generate dynamic QR code using PHP
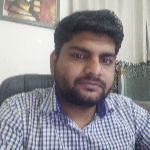
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website”. He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone, e27.co