For this purpose you need to do some optimization with image files. Image resize while uploading is one of them. All major website do it in the same way. If a user uploads a 5mb image file they resize it in different sizes and store on there server. It helps them to seed up there website and reduce there budget. Now take a look, how we can do it ourself.
PHP and HTML(index.php)
This file holds the basic of file uploading html markup and simple php upload handler
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
<?php include( 'function.php'); // settings $max_file_size = 1024*200; // 200kb $valid_exts = array('jpeg', 'jpg', 'png', 'gif'); // thumbnail sizes $sizes = array(100 => 100, 150 => 150, 250 => 250); if ($_SERVER['REQUEST_METHOD'] == 'POST' AND isset($_FILES['image'])) { if( $_FILES['image']['size'] < $max_file_size ){ // get file extension $ext = strtolower(pathinfo($_FILES['image']['name'], PATHINFO_EXTENSION)); if (in_array($ext, $valid_exts)) { /* resize image */ foreach ($sizes as $w => $h) { $files[] = resize($w, $h); } } else { $msg = 'Unsupported file'; } } else{ $msg = 'Please upload image smaller than 200KB'; } } ?> <html> <head> <title>Image resize while uploadin</title> <head> <body> <!-- file uploading form --> <form action="" method="post" enctype="multipart/form-data"> <label> <span>Choose image</span> <input type="file" name="image" accept="image/*" /> </label> <input type="submit" value="Upload" /> </form> </body> </html> |
Resize Function(function.php):
Resize function is based on PHP
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
/** * Image resize * @param int $width * @param int $height */ function resize($width, $height){ /* Get original image x y*/ list($w, $h) = getimagesize($_FILES['image']['tmp_name']); /* calculate new image size with ratio */ $ratio = max($width/$w, $height/$h); $h = ceil($height / $ratio); $x = ($w - $width / $ratio) / 2; $w = ceil($width / $ratio); /* new file name */ $path = 'uploads/'.$width.'x'.$height.'_'.$_FILES['image']['name']; /* read binary data from image file */ $imgString = file_get_contents($_FILES['image']['tmp_name']); /* create image from string */ $image = imagecreatefromstring($imgString); $tmp = imagecreatetruecolor($width, $height); imagecopyresampled($tmp, $image, 0, 0, $x, 0, $width, $height, $w, $h); /* Save image */ switch ($_FILES['image']['type']) { case 'image/jpeg': imagejpeg($tmp, $path, 100); break; case 'image/png': imagepng($tmp, $path, 0); break; case 'image/gif': imagegif($tmp, $path); break; default: exit; break; } return $path; /* cleanup memory */ imagedestroy($image); imagedestroy($tmp); } |
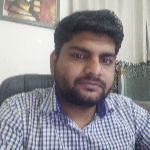
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website”. He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone, e27.co