Learn How to save or insert HTML Form records into Mongodb Database table using express js and mongoose js. In this article video you will learn about mongodb, express js and EJS template view engine. To insert a single record or document to MongoDB, you need to call save() method on document instance. Callback function(err, document) is an optional argument to save() method. Insertion happens asynchronously and any operations dependent on the inserted document has to happen in callback function for correctness.
For save HTML Form record to mongodb, you need to create HTML form in views folder file and define POST route in your index.js route file. Copy and Paste below html form code and route post method code :
Create index.ejs file with HTML Form:
Create index.js file in Views Directory and copy and paste below code.
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 |
<div class="container"> <div class="row"> <div id="signupbox" style="margin-top:50px" class="mainbox col-md-6 col-md-offset-3 col-sm-8 col-sm-offset-2"> <div class="panel panel-info"> <div class="panel-heading"> <div class="panel-title">Employee Details Form</div> </div> <div class="panel-body" > <form action="/" id="EmployeeForm" class="form-horizontal" method="post" role="form"> <div id="signupalert" style="display:none" class="alert alert-danger"> <p>Error:</p> <span></span> </div> <div class="form-group"> <label for="firstname" class="col-md-3 control-label">Name</label> <div class="col-md-9"> <input type="text" class="form-control" name="uname" placeholder="Enter Your Name"> </div> </div> <div class="form-group"> <label for="email" class="col-md-3 control-label">Email</label> <div class="col-md-9"> <input type="text" class="form-control" name="email" placeholder="Email Address"> </div> </div> <div class="form-group"> <label for="lastname" class="col-md-3 control-label">Employee Type</label> <div class="col-md-9"> <select class="form-control" name="emptype"> <option value="Hourly">Hourly</option> <option value="Fixed">Fixed</option> </select> </div> </div> <div class="form-group"> <label for="password" class="col-md-3 control-label">Hourly Rate</label> <div class="col-md-9"> <input type="text" class="form-control" name="hrlyrate" placeholder="Enter Hourly Rate"> </div> </div> <div class="form-group"> <label for="icode" class="col-md-3 control-label"> Total Hour</label> <div class="col-md-9"> <input type="text" class="form-control" name="ttlhr" placeholder="Enter Total Hours"> </div> </div> <div class="form-group"> <!-- Button --> <div class="col-md-offset-3 col-md-9"> <input type="submit" name="submit" value="submit" class="btn btn-primary"> </div> </div> </form> </div> </div> </div> </div> </div> |
Define router.post(“/”) in index.js file
Copy and paste the below code in index.js file of routes folder.
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
const mongoose = require('mongoose'); mongoose.connect('mongodb://localhost:27017/employee', {useNewUrlParser: true}); var conn =mongoose.Collection; var employeeSchema =new mongoose.Schema({ name: String, email: String, etype: String, hourlyrate: Number, totalHour: Number, total: Number, }); var empModel = mongoose.model('Employee', employeeSchema); var employee =empModel.find({}); router.post('/', function(req, res, next) { var empDetails = new empModel({ name: req.body.uname, email: req.body.email, etype: req.body.emptype, hourlyrate: req.body.hrlyrate, totalHour: req.body.ttlhr, total: parseInt(req.body.hrlyrate) * parseInt(req.body.ttlhr), }); empDetails.save(function(err,req1){ if(err) throw err; employee.exec(function(err,data){ if(err) throw err; res.render('index', { title: 'Employee Records', records:data, success:'Record Inserted Successfully' }); }); }) }); |
You need to watch below video tutorials to get complete knowledge of above code:
If you will like our video or if you think this video is useful for you the please view and subscribe our YouTube channel for latest video updates. So don’t forget to click bell icon after subscribe our channel.
Follow Tutorialswebsite:
https://www.facebook.com/tutorialswebsite
https://twitter.com/techwebsitetrix
Filter Records from Database with HTML Filter Form using Express js and Mongoosejs
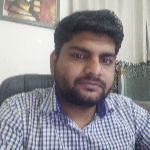
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website”. He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone, e27.co